Overview
When using ESP32, we investigated which illuminance sensor is good.
I understand the structure of the illuminance sensor, circuit diagrams, electronic work, and programming.
We are confirming whether it can be used for lux measurement using ESP32 and how it can be used for illuminance.
Video
Japanese【日本語】
English
Sensor List
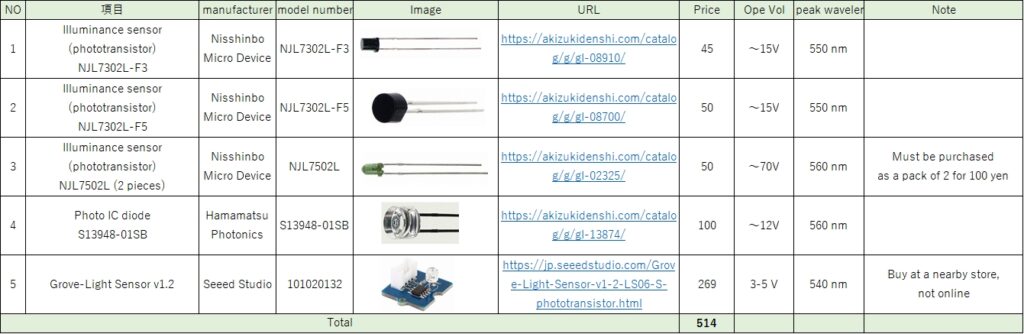
Cadmium sulfide (CdS) cells are cheap and easy to use (light can be treated as resistance).
However, cadmium seems to be a regulated element under the RoHS Directive (Rose Directive: European Union (EU) Directive on Restrictions on the Use of Certain Hazardous Substances in Electronic and Electrical Equipment).
It seems that the environmental load is large, so it was excluded from the selection.
Circuit
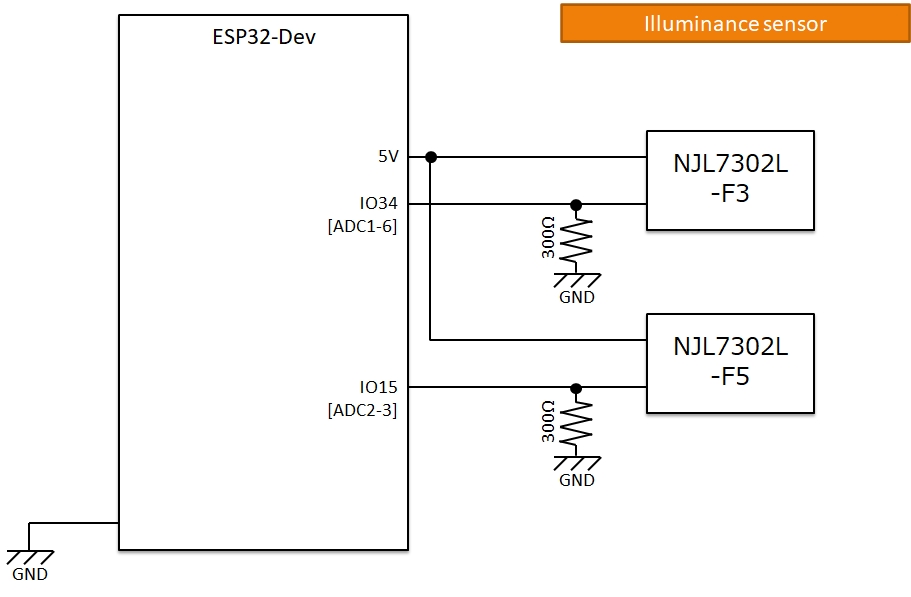
Program
//*************************************************************************
// Illuminance1 Ver2023.4.4
// Arduino board : ESP32(Arduino core for the ESP32) by Espressif Systems ver 2.0.7
// Written by IT-Taro
//***********************************************************************
#define ADC1_PIN 34
#define ADC2_PIN 15
unsigned long loopCount = 0;
void setup() {
// Serial monitor
Serial.begin(115200);
// ADC Setting
//analogSetAttenuation(ADC_0db); // ATT 0dB[Default 11db]
//analogSetAttenuation(ADC_6db); // ATT -6dB[Default 11db],
//analogSetWidth(10); // Resolution Default:12bit(0-4095)[9-12]
pinMode(ADC1_PIN, ANALOG);
pinMode(ADC2_PIN, ANALOG);
// Display Serial monitor
Serial.println("Setup completed!");
}
void loop() {
// Sleep[5 sec]
delay(5000);
loopCount++;
// ADC1[NJL7302L-F3]
uint16_t analog1_adc = analogRead(ADC1_PIN);
uint32_t analog1_mv = analogReadMilliVolts(ADC1_PIN);
// Convert voltage to current (I=V/R) [micro A (* 1000)]
double lightCur1 = (double)analog1_mv * 1000.0 / 300.0;
// Convert current to lux
double lightLux1 = pow(10, ((log10(lightCur1)-log10(1.8))/1.01399) );
Serial.printf("[%ld] NJL7302L-F3 ADC=%d, mV=%d[mV], lightCur=%5.2f[A], lightLux=%6.1f[lux]\n", loopCount, analog1_adc, analog1_mv, lightCur1, lightLux1);
// ADC2[NJL7302L-F5]
uint16_t analog2_adc = analogRead(ADC2_PIN);
uint32_t analog2_mv = analogReadMilliVolts(ADC2_PIN);
// Convert voltage to current (I=V/R)
double lightCur2 = (double)analog2_mv * 1000.0 / 300.0;
// Convert current to lux
double lightLux2 = pow(10, ((log10(lightCur2)-log10(1.8))/1.01399) );
Serial.printf("[%ld] NJL7302L-F5 ADC=%d, mV=%d[mV], lightCur=%5.2f[A], lightLux=%6.1f[lux]\n", loopCount, analog2_adc, analog2_mv, lightCur2, lightLux2);
}
//*************************************************************************
// Illuminance2 Ver2023.4.4
// Arduino board : ESP32(Arduino core for the ESP32) by Espressif Systems ver 2.0.7
// Written by IT-Taro
//***********************************************************************
#define ADC1_PIN 34
#define ADC2_PIN 15
unsigned long loopCount = 0;
void setup() {
// Serial monitor
Serial.begin(115200);
// ADC Setting
//analogSetAttenuation(ADC_0db); // ATT 0dB[Default 11db]
//analogSetAttenuation(ADC_6db); // ATT -6dB[Default 11db],
//analogSetWidth(10); // Resolution Default:12bit(0-4095)[9-12]
pinMode(ADC1_PIN, ANALOG);
pinMode(ADC2_PIN, ANALOG);
// Display Serial monitor
Serial.println("Setup completed!");
}
void loop() {
// Sleep[5 sec]
delay(5000);
loopCount++;
// ADC1[NJL7502L]
uint16_t analog1_adc = analogRead(ADC1_PIN);
uint32_t analog1_mv = analogReadMilliVolts(ADC1_PIN);
// Convert voltage to current (I=V/R) [micro A (* 1000)]
double lightCur1 = (double)analog1_mv * 1000.0 / 300.0;
// Convert current to lux
double lightLux1 = pow(10, ((log10(lightCur1)-log10(0.47))/0.99592) );
Serial.printf("[%ld] NJL7502L ADC=%d, mV=%d[mV], lightCur=%5.2f[A], lightLux=%6.1f[lux]\n", loopCount, analog1_adc, analog1_mv, lightCur1, lightLux1);
// ADC2[S13948]
uint16_t analog2_adc = analogRead(ADC2_PIN);
uint32_t analog2_mv = analogReadMilliVolts(ADC2_PIN);
// Convert voltage to current (I=V/R)
double lightCur2 = (double)analog2_mv * 1000.0 / 300.0;
// Convert current to lux
double lightLux2 = pow(10, ((log10(lightCur2)-log10(3.1))/0.98835) );
Serial.printf("[%ld] S13948 ADC=%d, mV=%d[mV], lightCur=%5.2f[A], lightLux=%6.1f[lux]\n", loopCount, analog2_adc, analog2_mv, lightCur2, lightLux2);
}
//*************************************************************************
// Illuminance3 Ver2023.4.4
// Arduino board : ESP32(Arduino core for the ESP32) by Espressif Systems ver 2.0.7
// Written by IT-Taro
//***********************************************************************
#define ADC1_PIN 36
unsigned long loopCount = 0;
void setup() {
// Serial monitor
Serial.begin(115200);
// ADC Setting
pinMode(ADC1_PIN, ANALOG);
}
void loop() {
// Sleep[5 sec]
delay(5000);
loopCount++;
// Check ADC
uint16_t analog1_adc = analogRead(ADC1_PIN);
uint32_t analog1_mv = analogReadMilliVolts(ADC1_PIN);
Serial.printf("[%ld] Grove Light ADC=%d, mV=%d[mV]\n", loopCount, analog1_adc, analog1_mv);
}
Measurement result
Measurement result 1 (5V, 330Ω)
When using a 330-ohm resistor, it is used when measuring a large brightness.
(However, the Grove sensor has a built-in resistance and cannot be changed. It cannot measure more than 5000 lux and is for indoor environments.)
Voltage can be measured by reflecting brightness, but lux measurement cannot be measured
because there is a difference of several times.
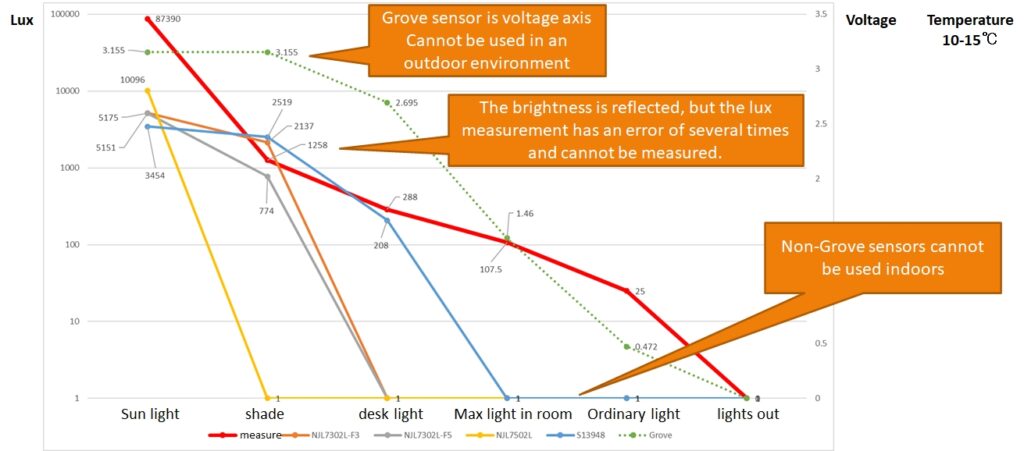
Measurement result 2 (5V, 10kΩ)
Just to make sure, I investigated whether lux measurement is possible.
(Originally, it cannot be used because it exceeds 3.9V)
Lux measurement is not possible due to an error of an order of magnitude. (Brightness can be reflected)
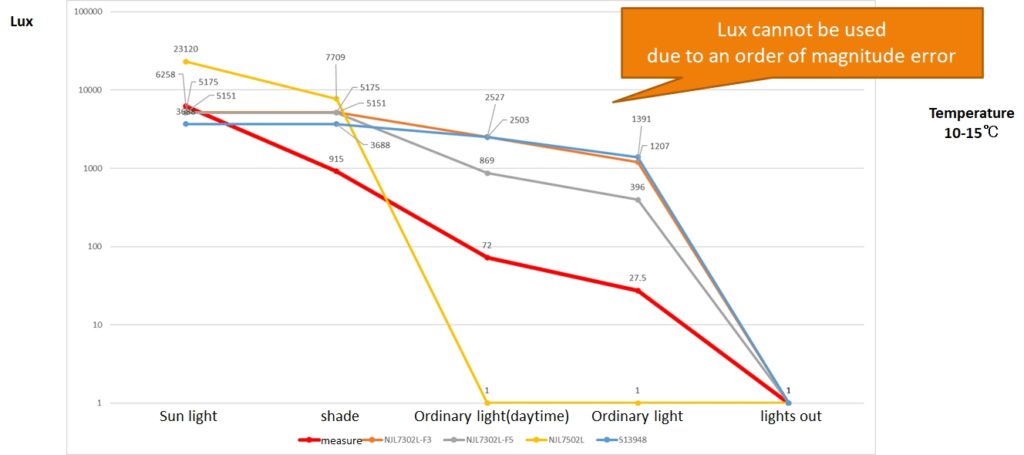
Measurement result 3 (3.3V, 10KΩ)
Using an input voltage of 3.3V and a resistance of 10KΩ, it is possible to determine ON/OFF of the lighting in the room (other than NJL7502L)
However, it is desirable to use it when installing in a bright place because the value is low even in a relatively bright place.
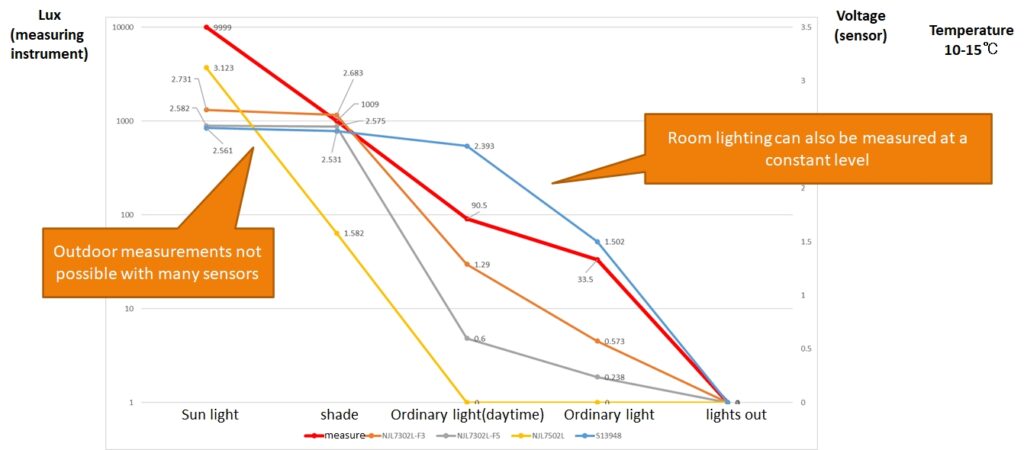
Measurement result 4 (3.3V, 200KΩ)
Using an input voltage of 3.3V and a resistance of 10KΩ, it is possible to determine whether the lighting in the room is ON/OFF.
Can be used when you want to determine whether the lighting is on or off in a dark place, such as when installed in the corner of a room.
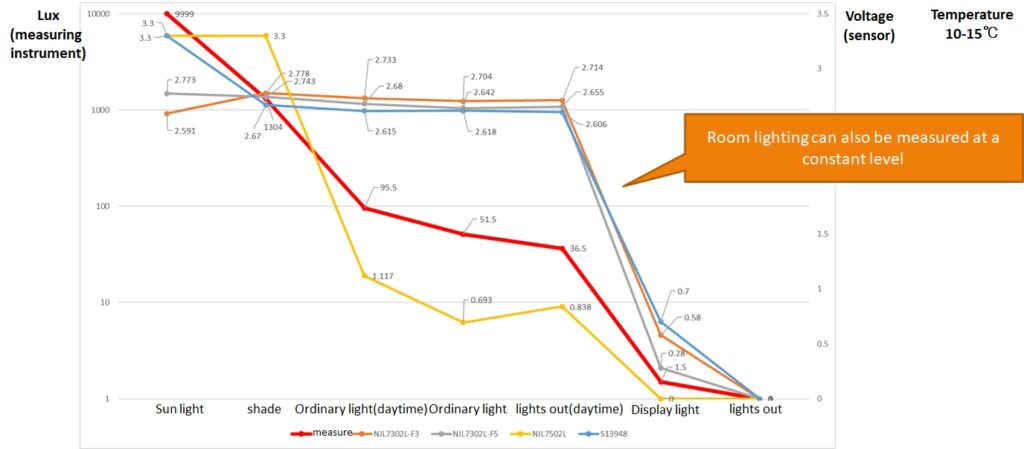
Document
Japanese【日本語】
English
Comments