In the Video
Japanese【日本語】
English【英語】
1. Overview
We will implement Wi-Fi connection, web server function, and SPIFFS on ESP32.
Although it is a series that eventually produces smart remote controls, this content alone is complete.
For development, we will use Arduino and ESP32 to realize it.
2. Overall flow of Smart Remote Controller Production
We will eventually create a smart remote control, and we will distribute it in a total of 7 posts.
This is the fifth “LED operation with a smartphone”.
No | Item | Content | Hard | Soft | Note |
---|---|---|---|---|---|
1 | Overall flow, system configuration, items used, reasons for selection, development environment, etc. | – | – | Another Post | |
2 | Green LED | Learn the basics for beginners. We will make “L blinking” that lights up and blinks the LED. | 〇 | 〇 | |
3 | Infrared receiving sensor | Description of infrared receiving sensor Schematic to Wiring, Software | 〇 | 〇 | |
4 | Infrared transmission LED | Infrared transmission LED description Schematic to Wiring, Software | 〇 | 〇 | |
5 | LED operation with smartphone(at home) | We will create software to operate the LED with smartphone. (Web server function, SPIFFS operation) | – | 〇 | This Post |
6 | Remote control with smartphone(at home) | We will create software that to operate the remote control with smartphone indoors. (Button name, signal save/read) | – | 〇 | Another Post |
7 | Operate from outside And AI speaker cooperation | We will create software to operate the remote control with smartphone from the outdoors, and AI speaker cooperation. | – | 〇 |
3. The development environment
Arduino was developed in Italy under the philosophy of “making things easier and easier to understand”.
Currently, it is widely used for learning all over the world, and the library is also substantial.
So, if you want to start electronic work, I think this is the only development environment.
Therefore, I use Arduino at this time.
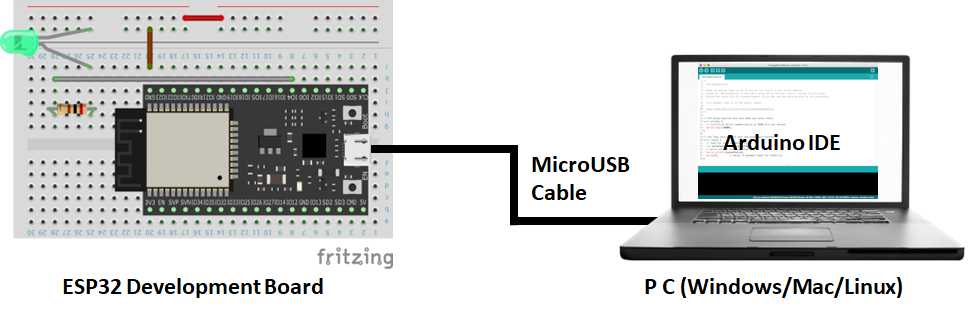
4-1. Software [String ver]
Here is the Arduino sketch(program).
//*************************************************************************
// Web LED Control Ver2023.1.26
// Arduino board : ESP32(Arduino core for the ESP32) by Espressif Systems ver 2.0.6
// Written by IT-Taro
//***********************************************************************
// Load the library
#include <ESPAsyncWebServer.h>
// Pin settings
const byte LED_PIN = 22; // green LED
bool ledFlag = true; // LED control flag
// Wi-Fi settings
const char *ssid = "##### SSID #####";
const char *password = "### PASSWORD ###";
IPAddress ip(192, 168, 1, 123); // IP address (IP used by this machine)
IPAddress gateway(192, 168, 1, 1); // default gateway
IPAddress subnet(255, 255, 255, 0); // sub-net mask
// Define WebServer (use port 80)
AsyncWebServer webServer ( 80 );
void setup(void) {
// Serial settings
Serial.begin(115200);
Serial.println ( );
// LED setting
pinMode ( LED_PIN, OUTPUT );
// Wireless Wi-Fi connection
WiFi.config( ip, gateway, subnet );
WiFi.begin ( ssid, password );
// Wi-Fi connection processing (infinite loop until connected)
while ( WiFi.status() != WL_CONNECTED ) {
// Blink the LED every 1 second
ledFlag = !ledFlag;
digitalWrite(LED_PIN, ledFlag);
// Wait for 1 second
delay ( 1000 );
Serial.print ( "." );
}
// Since the Wi-Fi connection was established, the IP address is displayed on the serial monitor
Serial.print ( "Wi-Fi Connected! IP address: " );
Serial.println ( WiFi.localIP() );
// LED lighting (Wi-Fi connection status)
digitalWrite ( LED_PIN, true );
// Set WebServer reception process "/"
webServer.on("/", HTTP_GET, [](AsyncWebServerRequest *request){
sendHtml(request); // Send web page content
Serial.println ( "TOP page" );
});
// Set WebServer reception processing "/on"
webServer.on("/on", HTTP_GET, [](AsyncWebServerRequest *request){
digitalWrite(LED_PIN, HIGH); // LED is lit by setting the LED pin to HIGH
Serial.println ( "LED ON" );
sendHtml(request); // Send web page content
});
// Set WebServer reception processing "/off"
webServer.on("/off", HTTP_GET, [](AsyncWebServerRequest *request){
digitalWrite(LED_PIN, LOW); // LED is lit by setting the LED pin to HIGH
Serial.println ( "LED OFF" );
sendHtml(request); // Send web page content
});
// WebServer startup processing
webServer.begin();
Serial.println ( "Web server started" );
}
void loop(void){
}
// Send web page content (HTML)
void sendHtml(AsyncWebServerRequest *request) {
// Set the HTML (character string) to reply
String res_html ="\
<html>\r\n\
<center>\r\n\
<h1>LED Control</h1>\r\n\
<p><button type='button' onclick='location.href=\"/on\"' style='width:700px;height:200px;'><font size=+5>LED-ON</font></button></p>\r\n\
<p><button type='button' onclick='location.href=\"/off\"' style='width:700px;height:200px;'><font size=+5>LED-OFF</font></button></p>\r\n\
</center>\r\n\
</html>";
// Reply HTML
request->send ( 200, "text/html", res_html );
Serial.println ( "send html text" );
}
4-1. Software [File ver]
//*************************************************************************
// Web LED Control [File] Ver2023.1.26
// Arduino board : ESP32(Arduino core for the ESP32) by Espressif Systems ver 2.0.6
// Written by IT-Taro
//***********************************************************************
// Load the library
#include <ESPAsyncWebServer.h>
#include <SPIFFS.h>
// Pin settings
const byte LED_PIN = 22; // green LED
bool ledFlag = true; // LED control flag
// Wi-Fi settings
const char *ssid = "##### SSID #####";
const char *password = "### PASSWORD ###";
IPAddress ip(192, 168, 1, 123); // IP address (IP used by this machine)
IPAddress gateway(192, 168, 1, 1); // default gateway
IPAddress subnet(255, 255, 255, 0); // sub-net mask
// Define WebServer (use port 80)
AsyncWebServer webServer ( 80 );
void setup(void) {
// Serial settings
Serial.begin(115200);
Serial.println ( );
// SPIFFS start
SPIFFS.begin();
// LED setting
pinMode ( LED_PIN, OUTPUT );
// Wireless Wi-Fi connection
WiFi.config( ip, gateway, subnet );
WiFi.begin ( ssid, password );
// Wi-Fi connection processing (infinite loop until connected)
while ( WiFi.status() != WL_CONNECTED ) {
// Blink the LED every 1 second
ledFlag = !ledFlag;
digitalWrite(LED_PIN, ledFlag);
// Wait for 1 second
delay ( 1000 );
Serial.print ( "." );
}
// Since the Wi-Fi connection was established, the IP address is displayed on the serial monitor
Serial.print ( "Wi-Fi Connected! IP address: " );
Serial.println ( WiFi.localIP() );
// LED lighting (Wi-Fi connection status)
digitalWrite ( LED_PIN, true );
// Set WebServer reception process "/"
webServer.on("/", HTTP_GET, [](AsyncWebServerRequest *request){
sendHtml(request); // Send web page content
Serial.println ( "TOP page" );
});
// Set WebServer reception processing "/on"
webServer.on("/on", HTTP_GET, [](AsyncWebServerRequest *request){
digitalWrite(LED_PIN, HIGH); // LED is lit by setting the LED pin to HIGH
Serial.println ( "LED ON" );
sendHtml(request); // Send web page content
});
// Set WebServer reception processing "/off"
webServer.on("/off", HTTP_GET, [](AsyncWebServerRequest *request){
digitalWrite(LED_PIN, LOW); // LED is lit by setting the LED pin to HIGH
Serial.println ( "LED OFF" );
sendHtml(request); // Send web page content
});
// WebServer startup processing
webServer.begin();
Serial.println ( "Web server started" );
}
void loop(void){
}
// Send web page content (HTML)
void sendHtml(AsyncWebServerRequest *request) {
// Send web page
request->send(SPIFFS, "/ledControl.html", "text/html");
Serial.println ( "send html file" );
}
<html>
<center>
<h1>LED Control [file]</h1>
<p><button type='button' onclick='location.href="/on"' style='width:700px;height:200px;'><font size=+5>LED-ON</font></button></p>
<p><button type='button' onclick='location.href="/off"' style='width:700px;height:200px;'><font size=+5>LED-OFF</font></button></p>
</center>
</html>
Comments